OverlaySplitView#
Added in version 1.4.
Superclasses: Widget
, InitiallyUnowned
, Object
Implemented Interfaces: Swipeable
, Accessible
, Buildable
, ConstraintTarget
A widget presenting sidebar and content side by side or as an overlay.
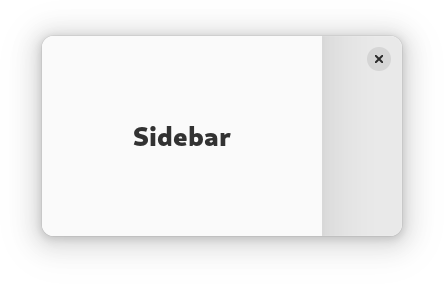
AdwOverlaySplitView
has two children: sidebar and content, and displays
them side by side.
When collapsed
is set to TRUE
, the sidebar is
instead shown as an overlay above the content widget.
The sidebar can be hidden or shown using the
show_sidebar
property.
Sidebar can be displayed before or after the content, this can be controlled
with the sidebar_position
property.
Collapsing the split view automatically hides the sidebar widget, and
uncollapsing it shows the sidebar. If this behavior is not desired, the
pin_sidebar
property can be used to override it.
AdwOverlaySplitView
supports an edge swipe gesture for showing the sidebar,
and a swipe from the sidebar for hiding it. Gestures are only supported on
touchscreen, but not touchpad. Gestures can be controlled with the
enable_show_gesture
and
enable_hide_gesture
properties.
See also NavigationSplitView
.
AdwOverlaySplitView
is typically used together with an Breakpoint
setting the collapsed
property to TRUE
on small widths, as follows:
<object class="AdwWindow">
<property name="default-width">800</property>
<property name="default-height">800</property>
<child>
<object class="AdwBreakpoint">
<condition>max-width: 400sp</condition>
<setter object="split_view" property="collapsed">True</setter>
</object>
</child>
<property name="content">
<object class="AdwOverlaySplitView" id="split_view">
<property name="sidebar">
<!-- ... -->
</property>
<property name="content">
<!-- ... -->
</property>
</object>
</property>
</object>
AdwOverlaySplitView
is often used for implementing the
utility pane
pattern.
Sizing#
When not collapsed, AdwOverlaySplitView
changes the sidebar width
depending on its own width.
If possible, it tries to allocate a fraction of the total width, controlled
with the sidebar_width_fraction
property.
The sidebar also has minimum and maximum sizes, controlled with the
min_sidebar_width
and
max_sidebar_width
properties.
The minimum and maximum sizes are using the length unit specified with the
sidebar_width_unit
.
By default, sidebar is using 25% of the total width, with 180sp as the minimum size and 280sp as the maximum size.
When collapsed, the preferred width fraction is ignored and the sidebar uses
max_sidebar_width
when possible.
Header Bar Integration#
When used inside AdwOverlaySplitView
, HeaderBar
will automatically
hide the window buttons in the middle.
The AdwOverlaySplitView
implementation of the Buildable
interface supports setting the sidebar widget by specifying “sidebar” as the
“type” attribute of a <child>
element, Specifying “content” child type or
omitting it results in setting the content widget.
CSS nodes#
AdwOverlaySplitView
has a single CSS node with the name
overlay-split-view
.
It contains two nodes with the name widget
, containing the sidebar and
content children.
When not collapsed, they have the .sidebar-view
and .content-view
style
classes respectively.
overlay-split-view
├── widget.sidebar-pane
│ ╰── [sidebar child]
╰── widget.content-pane
╰── [content child]
When collapsed, the one containing the sidebar child has the .background
style class and the other one has no style classes.
overlay-split-view
├── widget.background
│ ╰── [sidebar child]
╰── widget
╰── [content child]
Accessibility#
AdwOverlaySplitView
uses the GTK_ACCESSIBLE_ROLE_GROUP
role.
Constructors#
Methods#
- class OverlaySplitView
-
- get_enable_hide_gesture() bool #
Gets whether
self
can be closed with a swipe gesture.Added in version 1.4.
- get_enable_show_gesture() bool #
Gets whether
self
can be opened with an edge swipe gesture.Added in version 1.4.
- get_sidebar_width_fraction() float #
Gets the preferred sidebar width fraction for
self
.Added in version 1.4.
- get_sidebar_width_unit() LengthUnit #
Gets the length unit for minimum and maximum sidebar widths.
Added in version 1.4.
- set_collapsed(collapsed: bool) None #
Sets whether
self
view is collapsed.When collapsed, the sidebar widget is presented as an overlay above the content widget, otherwise they are displayed side by side.
Added in version 1.4.
- Parameters:
collapsed – whether
self
is collapsed
- set_content(content: Widget | None = None) None #
Sets the content widget for
self
.Added in version 1.4.
- Parameters:
content – the content widget
- set_enable_hide_gesture(enable_hide_gesture: bool) None #
Sets whether
self
can be closed with a swipe gesture.Only touchscreen swipes are supported.
Added in version 1.4.
- Parameters:
enable_hide_gesture – whether
self
can be closed with a swipe gesture
- set_enable_show_gesture(enable_show_gesture: bool) None #
Sets whether
self
can be opened with an edge swipe gesture.Only touchscreen swipes are supported.
Added in version 1.4.
- Parameters:
enable_show_gesture – whether
self
can be opened with a swipe gesture
- set_max_sidebar_width(width: float) None #
Sets the maximum sidebar width for
self
.Maximum width is affected by
sidebar_width_unit
.The sidebar widget can still be allocated with larger width if its own minimum width exceeds it.
Added in version 1.4.
- Parameters:
width – the maximum width
- set_min_sidebar_width(width: float) None #
Sets the minimum sidebar width for
self
.Minimum width is affected by
sidebar_width_unit
.The sidebar widget can still be allocated with larger width if its own minimum width exceeds it.
Added in version 1.4.
- Parameters:
width – the minimum width
- set_pin_sidebar(pin_sidebar: bool) None #
Sets whether the sidebar widget is pinned for
self
.By default, collapsing
self
automatically hides the sidebar widget, and uncollapsing it shows the sidebar. If set toTRUE
, sidebar visibility never changes on its own.Added in version 1.4.
- Parameters:
pin_sidebar – whether to pin the sidebar widget
- set_show_sidebar(show_sidebar: bool) None #
Sets whether the sidebar widget is shown for
self
.Added in version 1.4.
- Parameters:
show_sidebar – whether to show the sidebar widget
- set_sidebar(sidebar: Widget | None = None) None #
Sets the sidebar widget for
self
.Added in version 1.4.
- Parameters:
sidebar – the sidebar widget
- set_sidebar_position(position: PackType) None #
Sets the sidebar position for
self
.If it’s set to
GTK_PACK_START
, the sidebar is displayed before the content, ifGTK_PACK_END
, it’s displayed after the content.Added in version 1.4.
- Parameters:
position – the new position
- set_sidebar_width_fraction(fraction: float) None #
Sets the preferred sidebar width as a fraction of the total width of
self
.The preferred width is additionally limited by
min_sidebar_width
andmax_sidebar_width
.The sidebar widget can be allocated with larger width if its own minimum width exceeds the preferred width.
Added in version 1.4.
- Parameters:
fraction – the preferred width fraction
- set_sidebar_width_unit(unit: LengthUnit) None #
Sets the length unit for minimum and maximum sidebar widths.
See
min_sidebar_width
andmax_sidebar_width
.Added in version 1.4.
- Parameters:
unit – the length unit
Properties#
- class OverlaySplitView
-
- props.sidebar_width_unit: LengthUnit#
The type of the None singleton.
Added in version 1.4.