NavigationView#
Added in version 1.4.
Superclasses: Widget
, InitiallyUnowned
, Object
Implemented Interfaces: Swipeable
, Accessible
, Buildable
, ConstraintTarget
A page-based navigation container.
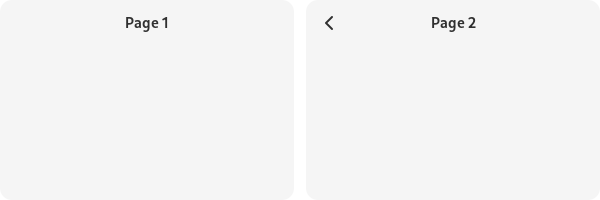
AdwNavigationView
presents one child at a time, similar to
Stack
.
AdwNavigationView
can only contain NavigationPage
children.
It maintains a navigation stack that can be controlled with
push
and pop
. The whole
navigation stack can also be replaced using replace
.
AdwNavigationView
allows to manage pages statically or dynamically.
Static pages can be added using the add
method. The
AdwNavigationView
will keep a reference to these pages, but they aren’t
accessible to the user until push
is called (except
for the first page, which is pushed automatically). Use the
remove
method to remove them. This is useful for
applications that have a small number of unique pages and just need
navigation between them.
Dynamic pages are automatically destroyed once they are popped off the
navigation stack. To add a page like this, push it using the
push
method without calling
add
first.
Tags#
Static pages, as well as any pages in the navigation stack, can be accessed
by their tag
. For example,
push_by_tag
can be used to push a static page that’s
not in the navigation stack without having to keep a reference to it manually.
Header Bar Integration#
When used inside AdwNavigationView
, HeaderBar
will automatically
display a back button that can be used to go back to the previous page when
possible. The button also has a context menu, allowing to pop multiple pages
at once, potentially across multiple navigation views.
Set show_back_button
to FALSE
to disable this behavior
in rare scenarios where it’s unwanted.
AdwHeaderBar
will also display the title of the AdwNavigationPage
it’s
placed into, so most applications shouldn’t need to customize it at all.
Shortcuts and Gestures#
AdwNavigationView
supports the following shortcuts for going to the
previous page:
Escape (unless
pop_on_escape
is set toFALSE
)Alt+:kbd:
←
Back mouse button
Additionally, it supports interactive gestures:
One-finger swipe towards the right on touchscreens
Scrolling towards the right on touchpads (usually two-finger swipe)
These gestures have transitions enabled regardless of the
animate_transitions
value.
Applications can also enable shortcuts for pushing another page onto the
navigation stack via connecting to the get_next_page
signal, in that case the following shortcuts are supported:
Alt+:kbd:
→
Forward mouse button
Swipe/scrolling towards the left
For right-to-left locales, the gestures and shortcuts are reversed.
can_pop
can be used to disable them, along with the
header bar back buttons.
Actions#
AdwNavigationView
defines actions for controlling the navigation stack.
actions for controlling the navigation stack:
navigation.push
takes a string parameter specifying the tag of the page to
push, and is equivalent to calling push_by_tag
.
navigation.pop
doesn’t take any parameters and pops the current page from
the navigation stack, equivalent to calling pop
.
AdwNavigationView
allows to add pages as children, equivalent to using the
add
method.
Example of an AdwNavigationView
UI definition:
<object class="AdwNavigationView">
<child>
<object class="AdwNavigationPage">
<property name="title" translatable="yes">Page 1</property>
<property name="child">
<object class="AdwToolbarView">
<child type="top">
<object class="AdwHeaderBar"/>
</child>
<property name="content">
<object class="GtkButton">
<property name="label" translatable="yes">Open Page 2</property>
<property name="halign">center</property>
<property name="valign">center</property>
<property name="action-name">navigation.push</property>
<property name="action-target">'page-2'</property>
<style>
<class name="pill"/>
</style>
</object>
</property>
</object>
</property>
</object>
</child>
<child>
<object class="AdwNavigationPage">
<property name="title" translatable="yes">Page 2</property>
<property name="tag">page-2</property>
<property name="child">
<object class="AdwToolbarView">
<child type="top">
<object class="AdwHeaderBar"/>
</child>
<property name="content">
<!-- ... -->
</property>
</object>
</property>
</object>
</child>
</object>
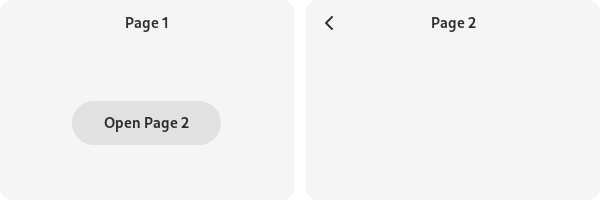
CSS nodes#
AdwNavigationView
has a single CSS node with the name navigation-view
.
Accessibility#
AdwNavigationView
uses the GTK_ACCESSIBLE_ROLE_GROUP
role.
Constructors#
Methods#
- class NavigationView
- add(page: NavigationPage) → None#
Permanently adds
page
toself
.Any page that has been added will stay in
self
even after being popped from the navigation stack.Adding a page while no page is visible will automatically push it to the navigation stack.
See
remove
.Added in version 1.4.
- Parameters:
page – the page to add
- find_page(tag: str) → NavigationPage | None#
Finds a page in
self
by its tag.See
tag
.Added in version 1.4.
- Parameters:
tag – a page tag
- get_animate_transitions() → bool#
Gets whether
self
animates page transitions.Added in version 1.4.
- get_navigation_stack() → ListModel#
Returns a
ListModel
that contains the pages in navigation stack.The pages are sorted from root page to visible page.
This can be used to keep an up-to-date view.
Added in version 1.4.
- get_pop_on_escape() → bool#
Gets whether pressing Escape pops the current page on
self
.Added in version 1.4.
- get_previous_page(page: NavigationPage) → NavigationPage | None#
Gets the previous page for
page
.If
page
is in the navigation stack, returns the page poppingpage
will reveal.If
page
is the root page or is not in the navigation stack, returnsNULL
.Added in version 1.4.
- Parameters:
page – a page in
self
- get_visible_page() → NavigationPage | None#
Gets the currently visible page in
self
.Added in version 1.4.
- pop() → bool#
Pops the visible page from the navigation stack.
Does nothing if the navigation stack contains less than two pages.
If
add
hasn’t been called, the page is automatically removed.popped
will be emitted for the current visible page.See
pop_to_page
andpop_to_tag
.Added in version 1.4.
- pop_to_page(page: NavigationPage) → bool#
Pops pages from the navigation stack until
page
is visible.page
must be in the navigation stack.If
add
hasn’t been called for any of the popped pages, they are automatically removed.popped
will be be emitted for each of the popped pages.See
pop
andpop_to_tag
.Added in version 1.4.
- Parameters:
page – the page to pop to
- pop_to_tag(tag: str) → bool#
Pops pages from the navigation stack until page with the tag
tag
is visible.The page must be in the navigation stack.
If
add
hasn’t been called for any of the popped pages, they are automatically removed.popped
will be emitted for each of the popped pages.See
pop_to_page
andtag
.Added in version 1.4.
- Parameters:
tag – a page tag
- push(page: NavigationPage) → None#
Pushes
page
onto the navigation stack.If
add
hasn’t been called, the page is automatically removed once it’s popped.pushed
will be emitted forpage
.See
push_by_tag
.Added in version 1.4.
- Parameters:
page – the page to push
- push_by_tag(tag: str) → None#
Pushes the page with the tag
tag
onto the navigation stack.If
add
hasn’t been called, the page is automatically removed once it’s popped.pushed
will be emitted for the page.See
push
andtag
.Added in version 1.4.
- Parameters:
tag – the page tag
- remove(page: NavigationPage) → None#
Removes
page
fromself
.If
page
is currently in the navigation stack, it will be removed once it’s popped. Otherwise, it’s removed immediately.See
add
.Added in version 1.4.
- Parameters:
page – the page to remove
- replace(pages: list[NavigationPage]) → None#
Replaces the current navigation stack with
pages
.The last page becomes the visible page.
Replacing the navigation stack has no animation.
If
add
hasn’t been called for any pages that are no longer in the navigation stack, they are automatically removed.n_pages
can be 0, in that case no page will be visible after calling this method. This can be useful for removing all pages fromself
.The
replaced
signal will be emitted.See
replace_with_tags
.Added in version 1.4.
- Parameters:
pages – the new navigation stack
- replace_with_tags(tags: list[str]) → None#
Replaces the current navigation stack with pages with the tags
tags
.The last page becomes the visible page.
Replacing the navigation stack has no animation.
If
add
hasn’t been called for any pages that are no longer in the navigation stack, they are automatically removed.n_tags
can be 0, in that case no page will be visible after calling this method. This can be useful for removing all pages fromself
.The
replaced
signal will be emitted.See
replace
andtag
.Added in version 1.4.
- Parameters:
tags – tags of the pages in the navigation stack
Properties#
- class NavigationView
-
- props.visible_page: NavigationPage#
The type of the None singleton.
Added in version 1.4.
Signals#
- class NavigationView.signals
- get_next_page() → NavigationPage | None#
The type of the None singleton.
Added in version 1.4.
- popped(page: NavigationPage) → None#
The type of the None singleton.
Added in version 1.4.
- Parameters:
page – the popped page